Sunday 21 December 2014
Monday 22 September 2014
Sunday 21 September 2014
Wednesday 17 September 2014
Class 8 : Program
Visual Basic Programs
This is the same video I posted few days back, it was not very clear, I hope this is OK.
Tuesday 16 September 2014
Saturday 13 September 2014
Class 9 -Scanner
Input Method:
We are going to use Scanner for accepting values from the user . Its a simple one.
First include the package util.
import java.util.*;
Only small letters and * indicates all the files from the package and there is a semi colon in the end.
Then comes the main function
public static void main( )
{
Scanner S=new Scanner(System.in);
Remember S in red colour are capital letters. Here S is a variable can be in small or capital letter or any other variable name.
There are different statements for accepting different data type values.
int a= S.nextInt( );
float f= S.nextFloat( );
double x=S.nextDouble( );
But accepting character and string are different.
char ch=(char)System.in.read( );
String st=S.next( );
String st1=S.nextLine( );
next( ) accepts a word till a space and keeps the cursor in the same line.
nextLine( ) accepts words and moves the cursor to the next line after accepting the value.
Let us accept two numbers.
import java.util.*; ------------------------------------ 1
class ex1
{
public static void main( )
{
Scanner s=new Scanner(System.in); --------------- 2
int a, b,sum; --------------------------------------------- 3
System.out.println("Enter the first number"); ------ 4
a=s.nextInt( ); ------------------------------------------- 5
System.out.println("Enter the second number");----- 4
b=s.nextInt( );-------------------------------------------- 5
sum=a+b; -------------------------------------------------6
System.out.print("Total = " +sum);------------------- 7
}
}
1 is the first line in the program.
2 Write any variable instead of s , if you want.
3 is variable declaration.
4 is just a prompt message. A remainder to the user to enter a number.
5 Since I am accepting integer values, it should be nextInt. Remember no space after next and I in Int is capital.
6 is the calculation
7 is the output.
For other programs step 3 and step 5 will be different according to the data type you use.
We are going to use Scanner for accepting values from the user . Its a simple one.
First include the package util.
import java.util.*;
Only small letters and * indicates all the files from the package and there is a semi colon in the end.
Then comes the main function
public static void main( )
{
Scanner S=new Scanner(System.in);
Remember S in red colour are capital letters. Here S is a variable can be in small or capital letter or any other variable name.
There are different statements for accepting different data type values.
int a= S.nextInt( );
float f= S.nextFloat( );
double x=S.nextDouble( );
But accepting character and string are different.
char ch=(char)System.in.read( );
String st=S.next( );
String st1=S.nextLine( );
next( ) accepts a word till a space and keeps the cursor in the same line.
nextLine( ) accepts words and moves the cursor to the next line after accepting the value.
Let us accept two numbers.
import java.util.*; ------------------------------------ 1
class ex1
{
public static void main( )
{
Scanner s=new Scanner(System.in); --------------- 2
int a, b,sum; --------------------------------------------- 3
System.out.println("Enter the first number"); ------ 4
a=s.nextInt( ); ------------------------------------------- 5
System.out.println("Enter the second number");----- 4
b=s.nextInt( );-------------------------------------------- 5
sum=a+b; -------------------------------------------------6
System.out.print("Total = " +sum);------------------- 7
}
}
1 is the first line in the program.
2 Write any variable instead of s , if you want.
3 is variable declaration.
4 is just a prompt message. A remainder to the user to enter a number.
5 Since I am accepting integer values, it should be nextInt. Remember no space after next and I in Int is capital.
6 is the calculation
7 is the output.
For other programs step 3 and step 5 will be different according to the data type you use.
Tuesday 9 September 2014
Class 8
Declaring a variable
The first line in the program would be variable declaration. It includes the Dim statement , name of the variable and the data type.
Dim age as integer
Dim name as String
If you have more than one variable,
Dim a,b,c as Integer (same data type)
Dim x,y as Integer, i,j as Double (different data types)
Now the second step.
We need to give values for those variables.
Dim a as Integer = 100
or
Dim A as Integer
A=10
Activity
1. Declare the integer variables x,y and z
Dim x,y,z as Integer
2. Assign values 5 to x, 10 to y and 15 to z
x=5
y=10
z=15
3. What are the values of C, N1, N2, N3 :
A=5
B=10
C=A+B
N1=A+10
N2=B
N3=5+N2
C=15( since A is 5 and B is 10)
N1=15 ( A is 5 and add 10 to it)
N2=10(B is 10)
N3=15 (N2 is 10 and add 5 to it)
Sring Functions
1. Print Len("Cotton Boys)
11(including space)
2. Print Left("BCBS", 2)
BC
3. Print Right("Bangalore",4)
lore
4. Print Mid("Sesquicentennial",7,4)
cent
5. Print Ucase("Sony")
SONY
6. Print Lcase("Computer SCIENCE")
computer science
7. Print Instr("Happiness","in")
5 (5 is the place value of i)
Print Instr("Happiness","at")
0 (since there is no at in the string Happiness)
8. Print val("40") + val("20")
60
Print ("40") + ("60")
4060( since both are NOT numbers but strings, so they are
joined together not added)
9. Print Replace("World/Wide","/","_")
World_Wide(/ is replaced with _)
The first line in the program would be variable declaration. It includes the Dim statement , name of the variable and the data type.
Dim age as integer
Dim name as String
If you have more than one variable,
Dim a,b,c as Integer (same data type)
Dim x,y as Integer, i,j as Double (different data types)
Now the second step.
We need to give values for those variables.
Dim a as Integer = 100
or
Dim A as Integer
A=10
Activity
1. Declare the integer variables x,y and z
Dim x,y,z as Integer
2. Assign values 5 to x, 10 to y and 15 to z
x=5
y=10
z=15
3. What are the values of C, N1, N2, N3 :
A=5
B=10
C=A+B
N1=A+10
N2=B
N3=5+N2
C=15( since A is 5 and B is 10)
N1=15 ( A is 5 and add 10 to it)
N2=10(B is 10)
N3=15 (N2 is 10 and add 5 to it)
Sring Functions
1. Print Len("Cotton Boys)
11(including space)
2. Print Left("BCBS", 2)
BC
3. Print Right("Bangalore",4)
lore
4. Print Mid("Sesquicentennial",7,4)
cent
5. Print Ucase("Sony")
SONY
6. Print Lcase("Computer SCIENCE")
computer science
7. Print Instr("Happiness","in")
5 (5 is the place value of i)
Print Instr("Happiness","at")
0 (since there is no at in the string Happiness)
8. Print val("40") + val("20")
60
Print ("40") + ("60")
4060( since both are NOT numbers but strings, so they are
joined together not added)
9. Print Replace("World/Wide","/","_")
World_Wide(/ is replaced with _)
Monday 8 September 2014
Class 7 - Hyperlink
Write a complete HTML program to create a webpage as given below.
The title of the page is an image file (title.png) , and the
other image file is gladiator.jpg.
other image file is gladiator.jpg.
The file names of different notepad files are histry.html, image1.html, image2.html, image3.html, image4.html. Link all the pages.Create only the main file.
Now you have to create only the main file.
<html>
<head>
<title> Cottonian Shield</title>
</head>
<body>
<center><img src=title.png></center> <br>
<center><img src=gladiator.jpg></center><br>
<a href=histry.html>History</a><br>
<a href=image1.html>Image 1</a><br>
<a href=image2.html>Image 2</a><br>
<a href=image3.html>Image 3</a><br>
<a href=image4.html>Image 4</a><br>
</body>
</html>
Remember after <a href write the file name if it is given in the question or else assume some name. Do not write the picture name there, write only the notepad file name, close the bracket. Then write the hypertext that is the text which on clicking will take you to the given page . In the example given below the hypertexts are History,Image 1,Image2,Image3,Image 4.
By the way those are my boys Jeswanth, Vinay and Sapthagiri (8 B last year). Now they are in senior team.
Now you have to create only the main file.
<html>
<head>
<title> Cottonian Shield</title>
</head>
<body>
<center><img src=title.png></center> <br>
<center><img src=gladiator.jpg></center><br>
<a href=histry.html>History</a><br>
<a href=image1.html>Image 1</a><br>
<a href=image2.html>Image 2</a><br>
<a href=image3.html>Image 3</a><br>
<a href=image4.html>Image 4</a><br>
</body>
</html>
Remember after <a href write the file name if it is given in the question or else assume some name. Do not write the picture name there, write only the notepad file name, close the bracket. Then write the hypertext that is the text which on clicking will take you to the given page . In the example given below the hypertexts are History,Image 1,Image2,Image3,Image 4.
By the way those are my boys Jeswanth, Vinay and Sapthagiri (8 B last year). Now they are in senior team.
Class 7 - Revision(HTML)
Select the correct answer:
2. Container element has both
14. The text between <title> and </title> will be displayed
1. Write a program in HTML to display the following :
My first program ---------------------------- 1
I am in class 7 -----------------------------2
Bishop Cotton Boys' School -----------------------------3
<html>
<head>
<title> Example 1</title>
</head>
<body >
<b> My first program</b> <br>
I am in <I> class 7 </I> <p>
<u> Bishop Cotton Boys' School</p>
</body>
</html>
The line1 is darker than the rest of the program. This is because of the bold tag. to go to the next line use the <br> tag.
In line 2 , only class 7 is slanting , so use italics tag for that.
There is a blank line between line 2 and line 3, so paragraph tag should be used. Even if you close <p> before <u> tag also will give same effect.
In line 3,a line is drawn below the whole sentence using <u> tag.
2. What would be the output for the following program:
<html>
<head>
<title> Example 2</title>
</head>
<body bgcolor=olive > ------------------1
<font color=white size=6 face= Mistral> ------------------2
Computers<br>Class 7
<p>
Cottonnian shield...................
</p>
<!- I am going to draw a line - > -----------------3
<hr size=5 > -----------------4
</font>
</body>
</html>
Line 1 : the background colour. The spelling is bgcolor.
Line 2 : font color makes the text and numbers to turn to that particular colour. face changes the default font name to the name given.
Line 3 : This is the comment line , which will not be displayed in the web browser.
Line 4: The horizontal line with size 5
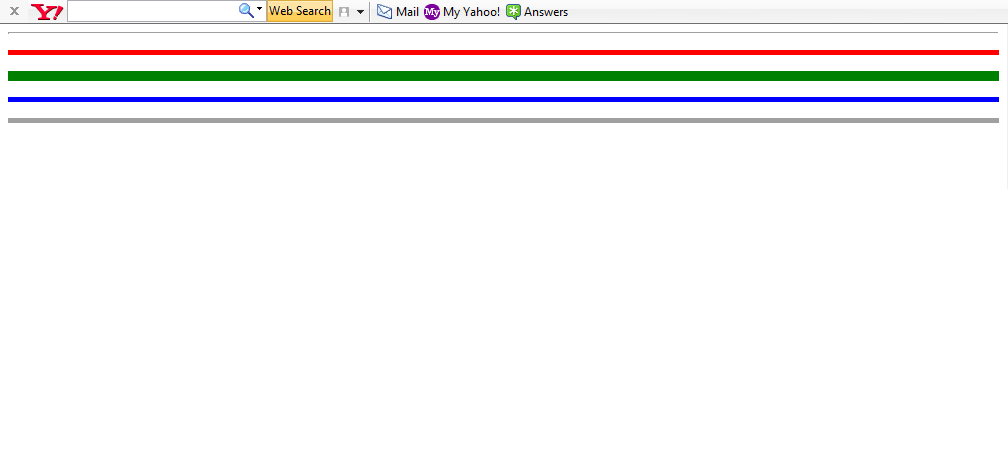
The above output has 5 lines. The first one is a thin line, because there is no size tag.
<html>
<head>
<title> Example 3</title>
</head>
<body>
<hr > <p>
<hr size=3 color=red> <p>
<hr size=8 color=green></p>
<hr size=3 align=right color=blue><p>
<hr size=3 noshade ></p>
</body>
</html>
Image
<img src=filename>
<img src= bird.jpg>
Attributes of image tag :
1. width - changes the width of the image
2. height - changes the height of the image
3. border - border around the image, default value is 0.
4. alt - if the image is not available, the alternate text will be displayed.
5. align - position of the image in the web page.
align =right/left/middle/top/bottom
<html>
<body>
<img src=moon.jpg height=400 width=400>
</body>
</html>
- HTML stands for
- Hyper Text Markup Language
- Hyper Text Makeup Language
2. Container element has both
- opening and middle tag
- opening and closing tag
- a line break tag
- an empty tag
- line break and an empty tag
- an editor
- a web browser
- search engine
- <body backcolor=red>
- <body bgcolour=red>
- <body bgcolor=red>
- <body backcolour=red>
- <body bg color=red>
- <text=green>
- <body textcolour=green>
- <body text=green>
- <textcolour=green>
- moves the cursor to the next line
- leaves a blank line
- leaves a paragraph
- comments
- title
- body
- <align=center>
- <align=centre>
- <p align=centre>
- <center>
- 1 to 7
- 1 to 6
- 1 to 3
- <dark>
- <b>
- <bold>
- <u>
- <line>
- <strike>
- <strikethrough>
- <head>
- <h1>
- <h6>
14. The text between <title> and </title> will be displayed
- in the title bar
- in the heading bar
- in the web page
- none of the above
- an additional information about the tag
- main tag
- an example
- MS Word
- Frontpage
- HTML
1. Write a program in HTML to display the following :
My first program ---------------------------- 1
I am in class 7 -----------------------------2
Bishop Cotton Boys' School -----------------------------3
<html>
<head>
<title> Example 1</title>
</head>
<body >
<b> My first program</b> <br>
I am in <I> class 7 </I> <p>
<u> Bishop Cotton Boys' School</p>
</body>
</html>
The line1 is darker than the rest of the program. This is because of the bold tag. to go to the next line use the <br> tag.
In line 2 , only class 7 is slanting , so use italics tag for that.
There is a blank line between line 2 and line 3, so paragraph tag should be used. Even if you close <p> before <u> tag also will give same effect.
In line 3,a line is drawn below the whole sentence using <u> tag.
2. What would be the output for the following program:
<html>
<head>
<title> Example 2</title>
</head>
<body bgcolor=olive > ------------------1
<font color=white size=6 face= Mistral> ------------------2
Computers<br>Class 7
<p>
Cottonnian shield...................
</p>
<!- I am going to draw a line - > -----------------3
<hr size=5 > -----------------4
</font>
</body>
</html>
Line 1 : the background colour. The spelling is bgcolor.
Line 2 : font color makes the text and numbers to turn to that particular colour. face changes the default font name to the name given.
Line 3 : This is the comment line , which will not be displayed in the web browser.
Line 4: The horizontal line with size 5
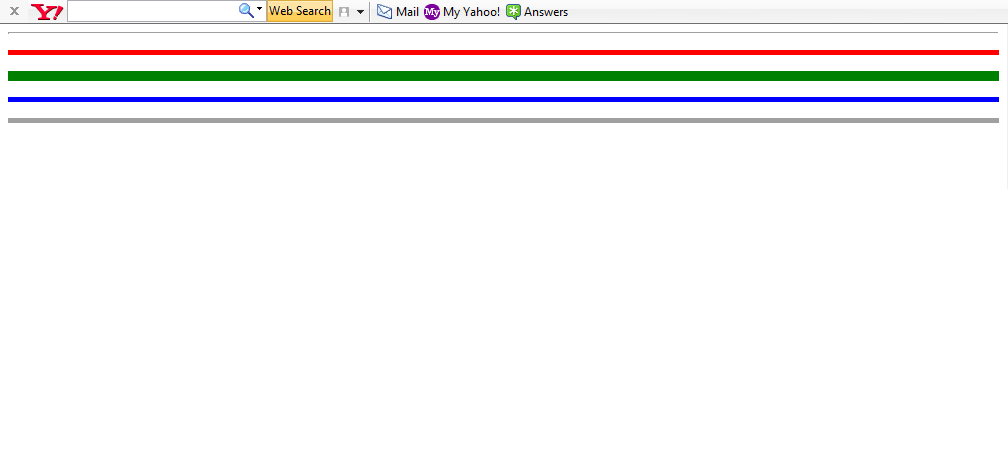
The above output has 5 lines. The first one is a thin line, because there is no size tag.
<html>
<head>
<title> Example 3</title>
</head>
<body>
<hr > <p>
<hr size=3 color=red> <p>
<hr size=8 color=green></p>
<hr size=3 align=right color=blue><p>
<hr size=3 noshade ></p>
</body>
</html>
Image
<img src=filename>
<img src= bird.jpg>
Attributes of image tag :
1. width - changes the width of the image
2. height - changes the height of the image
3. border - border around the image, default value is 0.
4. alt - if the image is not available, the alternate text will be displayed.
5. align - position of the image in the web page.
align =right/left/middle/top/bottom
<html>
<body>
<img src=moon.jpg height=400 width=400>
</body>
</html>
Thursday 28 August 2014
Tuesday 19 August 2014
Class 9 -Intro to JAVA programming
Creating a set of instructions to make the computer to do something is no Rocket Science. Just a bit of logic, bit of syntax, bit of patience, bit of interest and bit of concentration in the class are all you need to learn the language.
Programming , most of the times involve 4 steps:
Write a program to print your name and school name.
class prog1
{
public static void main( )
{
System.out.print("Kavitha");
System.out.print("Cottons");
}
}
This is the basic structure of the program. You might have noticed that the program did not use all the above 4 steps.
We don't have to use all the steps in all the programs.
The output is going to be :
KavithaCottons
System.out.print( ) displays anything written inside the brackets.
In case if I don't want the answer in single line , I have to use
System.out.println( ).
In case if I want the answer in a single line but separated by a space or a comma then,
System.out.print("Kavitha" + " " + "Cottons" ).
The answer will be Kavitha Cottons
In the print statement, the + symbol is not an addition symbol rather it combines two items, that is Kavitha and space and also space and Cottons. So there are 3 items Kavitha, space , Cottons.
So if I make a small change in my program the answers will be in two different lines.
class prog1
{
public static void main( )
{
System.out.println("Kavitha");
System.out.print("Cottons");
}
}
Example 2 :
Write a program to assign two values and print its sum.
class prog2
{
public static void main( )
{
int a, b,c; -------------------------------------- Step 1
a=5; ---------------------------------------Step 2
b=30; ----------------------------------------Step 2
c=a+b; --------------------------------------- Step 3
System.out.print(c); ------------------------- Step 4
}
}
The output will be 35
Step 1 : Declaration.
Mention the data types of the variables involved.
Step 2: Assign
Put some values in your variables.
Step 3 : Calculate
Add the values and store it in the new variable. Remember variable = constant not the other way round.
Step 4 : Print
Display the answer. you can also write
System.out.print("Sum= " + c);
The answer will be Sum = 35. This is the good way of displaying the answer with a message.
Steps 1 and 2 can be combined to form a single statement.
int a = 5, b=30, c;
Input Methods
We are going to learn 2 input methods .
Blue J Method
class prog3
{
public static void main( int a, int b ) ----- Accepting values from the user
{
int c;
c=a+b;
System.out.print("Sum = " + c);
}
}
Even though both a and b are of same data type , it should be mentioned twice and should be separated by comma(,).
public static void main( int a, b ) ------ Wrong
See you in the next post.
Programming , most of the times involve 4 steps:
- Declaration
- Assign / Input
- Calculation (if necessary)
Write a program to print your name and school name.
class prog1
{
public static void main( )
{
System.out.print("Kavitha");
System.out.print("Cottons");
}
}
This is the basic structure of the program. You might have noticed that the program did not use all the above 4 steps.
We don't have to use all the steps in all the programs.
The output is going to be :
KavithaCottons
System.out.print( ) displays anything written inside the brackets.
In case if I don't want the answer in single line , I have to use
System.out.println( ).
In case if I want the answer in a single line but separated by a space or a comma then,
System.out.print("Kavitha" + " " + "Cottons" ).
The answer will be Kavitha Cottons
In the print statement, the + symbol is not an addition symbol rather it combines two items, that is Kavitha and space and also space and Cottons. So there are 3 items Kavitha, space , Cottons.
So if I make a small change in my program the answers will be in two different lines.
class prog1
{
public static void main( )
{
System.out.println("Kavitha");
System.out.print("Cottons");
}
}
Example 2 :
Write a program to assign two values and print its sum.
class prog2
{
public static void main( )
{
int a, b,c; -------------------------------------- Step 1
a=5; ---------------------------------------Step 2
b=30; ----------------------------------------Step 2
c=a+b; --------------------------------------- Step 3
System.out.print(c); ------------------------- Step 4
}
}
The output will be 35
Step 1 : Declaration.
Mention the data types of the variables involved.
Step 2: Assign
Put some values in your variables.
Step 3 : Calculate
Add the values and store it in the new variable. Remember variable = constant not the other way round.
Step 4 : Print
Display the answer. you can also write
System.out.print("Sum= " + c);
The answer will be Sum = 35. This is the good way of displaying the answer with a message.
Steps 1 and 2 can be combined to form a single statement.
int a = 5, b=30, c;
Input Methods
We are going to learn 2 input methods .
- Blue J
- Scanner
Blue J Method
class prog3
{
public static void main( int a, int b ) ----- Accepting values from the user
{
int c;
c=a+b;
System.out.print("Sum = " + c);
}
}
Even though both a and b are of same data type , it should be mentioned twice and should be separated by comma(,).
public static void main( int a, b ) ------ Wrong
See you in the next post.
Thursday 14 August 2014
Class 10 (Array)
Array is an interesting topic. Imagine a situation where you
want to input the names of students in your class along with
the roll no and 3 subjects marks. There are 50 boys in your
class. Then how many variables you need? There is no point
in writing a program. Array comes in handy in such a
situation .
An array is a collection of values of same data type. Each element in an array is refered by the array name with the subscript or index.It is enclosed in square bracket [ ], whereas methods use ( ).
Like the normal declaration of variables, we need to declare the array also.
data type array name[];
int marks[];
Memory allocation:
data type array name[]=new datatype[size];
int a[]=new int[10];
How many values you are going to use should be mentioned where it is given size.The common mistake we make here is writing the variable name after new.
int a[]=new a[10]; is wrong
Initializing values
int a[]={10,20,30,40};
The place value of each element is called a subscript or an
index. The index value starts from zero, so the last
element index is one less than the size.
To find the length of an array use length.
int l=a.length;
No brackets after length. It finds the length of the array 'a'
which is 4 and it is stored in a variable l;
To accept 10 values from the user.
Example 1: Blue J method
class ar1
{
public static void main(int a[])
Example 2: Scanner Method
import java.util.*;
class ar1
{
public static void main()
{
int a[]=new int[10];--------------------------1
Scanner s=new Scanner(System.in);
int i,l,s=0;
l=a.length;------------------------------------2
System.out.println("Enter the values");
for(i=0;i<l;i++)------------------------------3
a[i]=s.nextInt();------------------------------4
for(i=0;i<l;i++)
s=s+a[i];-------------------------------------5
System.out.println(a[i]);
}
}
Step 1 : an array a is created ,
Step 2 :finds the Length
Step 3 : index starts from 0 and less than length l
Step 4 : The values are store in a.First value is stored in
a[0], second in a[1] and so on
Step 5: When i=0, a[0] value is added to s, when i=1 ,a[1] is added and so on..
Loop plays an important role in Arrays , whether it is for accepting values or printing it.
Accepting values :
Write the Java statements for the following:
Search
import java.util.*;
class linear{
public static void main()
{
int a[]=new int[10];
Scanner s=new Scanner(System.in);
int i,l,n,flag=0;
l=a.length ;
System.out.println("Enter the values");
for(i=0;i<l;i++)
a[i]=s.nextInt();
System.out.println("Enter the number to be searched");
n=s.nextInt();
for(i=0;i<l;i++)
{
if(a[i]==n)
{
flag=1;
break;
}
}
if(flag==1)
System.out.print(n+"found");
else
System.out.print(n+"not found");
}
}
Initially flag is 0, if the number is found the flag value will change to 1.
Binary Search
This might look little complicated,but if you understand how it works,it is a cake walk.
The prerequisite is that the array elements should be arranged in some order either in ascending or descending.
In the boards generally the question will not ask you to sort
and search in the same program.
The linear search takes more time since it has to check each
and every element sequentially.It is a good idea but not a great one.
So, here in binary search the idea is divide the array and discard whatever not necessary.
Step 1: we are going to divide the array into two parts.
For that we should find the middle value.
Step 2: Compare the number to be searched say 'n' with the middle value say 'm'.If both are same, Bingo.
Step 3: If the n is smaller than m, then you would find n in
the first half ,if the array is arranged in ascending order.Then
you dont want the second part anymore.discard it. So the
length changes after you discard the second part.Lower index
value remains the same that is 0, but upper limit becomes
middle value - 1.Then step 2 will be repeated.
If the n is bigger than m, then you would find n in the second
half ,if the array is arranged in ascending order.Then you
dont want the first part anymore.discard it. So the length
changes after you discard the second part.Upper index value
remains the same, but lower limit becomes middle value +
1.Then step 2 will be repeated.
Example :
The array is
14 , 23, 28, 36, 69, 88
The number to be searched is 23.
Step 1:
Middle value = (lower index +Upper index)/2
= (0+5)/2
= 2.5
Either round off or truncate it. Let us take 3.So the middle value is 36.
Step 2 :
Compare 23 and 3 rd element 36.Both are not same.
Step 3 :
23 is smaller than 36, that means 23 will not be there after 36.
so discard second half, that is elements after 36.Lower index is still 0, and upper index becomes the value before the middle value , i.e 28.
Checking will go on till it finds the value.
If the value to be searched is 88 then,
88 is bigger than 36, therefore 88 will not be in the list
before 36.
so discard first half, that is elements before 36.upper
index remains the same and lower index becomes the
value after the middle value.
Checking will go on till it finds the value. Otherwise it will print the nmber not in the list.
class binary
{
public static void main(int a[],int n)---------------------------1
{
int l,i,mid,place,flag=0;
l=a.length;--------------------------------------------------------2
int low=0,up= l -1;----------------------------------------------3
while(low <= upp)----------------------------------------------4
{
mid = (low+up)/2;------------------------------------------5
if(a[mid]==n)
{
flag=1;------------------------------------------------6
place=mid;--------------------------------------------7
break;-------------------------------------------------8
}
else if(a[mid] < n)-----------------------------------------9
low=mid+1;
else
upp=mid - 1;
}
if(flag==1)
System.out.print(n+"is found at+(place+1));-----------------10
else
System.out.print(n+"is not found ");
}
}
step 1: input the array, number to be searched
step 2: find the length of the array
step 3:initialize the values for lower and upper index. l-1 coz, index starts from 0.
step 4:the loop should continue as long as lower limit is less than upper limit.
step 5:find the middle value.common mistake here is
(a[low]+a[upp])/2
step 6:there is some variable with some intial value so that when we find the number, change that value.If the value is not changed then it means the number is not found,coz you would not have gone to step 6.
step 7: if you want to find exactly where the number occurs in the array,then assign the mid index, not mid value.It is not a[mid].
step 8:Once we found the number,come out. no more searching.
step 9:check the number with middle value , change the low or upper index accordingly.
Isn't that easy.
want to input the names of students in your class along with
the roll no and 3 subjects marks. There are 50 boys in your
class. Then how many variables you need? There is no point
in writing a program. Array comes in handy in such a
situation .
An array is a collection of values of same data type. Each element in an array is refered by the array name with the subscript or index.It is enclosed in square bracket [ ], whereas methods use ( ).
- Declaration
- Memory allocation
- Initialization
Like the normal declaration of variables, we need to declare the array also.
data type array name[];
int marks[];
Memory allocation:
data type array name[]=new datatype[size];
int a[]=new int[10];
How many values you are going to use should be mentioned where it is given size.The common mistake we make here is writing the variable name after new.
int a[]=new a[10]; is wrong
Initializing values
int a[]={10,20,30,40};
The place value of each element is called a subscript or an
index. The index value starts from zero, so the last
element index is one less than the size.
To find the length of an array use length.
int l=a.length;
No brackets after length. It finds the length of the array 'a'
which is 4 and it is stored in a variable l;
To accept 10 values from the user.
Example 1: Blue J method
class ar1
{
public static void main(int a[])
Example 2: Scanner Method
import java.util.*;
class ar1
{
public static void main()
{
int a[]=new int[10];--------------------------1
Scanner s=new Scanner(System.in);
int i,l,s=0;
l=a.length;------------------------------------2
System.out.println("Enter the values");
for(i=0;i<l;i++)------------------------------3
a[i]=s.nextInt();------------------------------4
for(i=0;i<l;i++)
s=s+a[i];-------------------------------------5
System.out.println(a[i]);
}
}
Step 1 : an array a is created ,
Step 2 :finds the Length
Step 3 : index starts from 0 and less than length l
Step 4 : The values are store in a.First value is stored in
a[0], second in a[1] and so on
Step 5: When i=0, a[0] value is added to s, when i=1 ,a[1] is added and so on..
Loop plays an important role in Arrays , whether it is for accepting values or printing it.
Accepting values :
- System.out.println("Enter the values");
for(i=0;i<l;i++)
a[i]=s.nextInt();
Printing :
for(i=0;i<l;i++)
System.out.println(a[i]);
Write the Java statements for the following:
- Declare 5 integers in code.
- Declare to store 10 names.
- Assign the values 10.5, 20.5, 30.5, 40.5 to an array number.
Search
- Linear
- Binary
import java.util.*;
class linear{
public static void main()
{
int a[]=new int[10];
Scanner s=new Scanner(System.in);
int i,l,n,flag=0;
l=a.length ;
System.out.println("Enter the values");
for(i=0;i<l;i++)
a[i]=s.nextInt();
System.out.println("Enter the number to be searched");
n=s.nextInt();
for(i=0;i<l;i++)
{
if(a[i]==n)
{
flag=1;
break;
}
}
if(flag==1)
System.out.print(n+"found");
else
System.out.print(n+"not found");
}
}
Initially flag is 0, if the number is found the flag value will change to 1.
Binary Search
This might look little complicated,but if you understand how it works,it is a cake walk.
The prerequisite is that the array elements should be arranged in some order either in ascending or descending.
In the boards generally the question will not ask you to sort
and search in the same program.
The linear search takes more time since it has to check each
and every element sequentially.It is a good idea but not a great one.
So, here in binary search the idea is divide the array and discard whatever not necessary.
Step 1: we are going to divide the array into two parts.
For that we should find the middle value.
Step 2: Compare the number to be searched say 'n' with the middle value say 'm'.If both are same, Bingo.
Step 3: If the n is smaller than m, then you would find n in
the first half ,if the array is arranged in ascending order.Then
you dont want the second part anymore.discard it. So the
length changes after you discard the second part.Lower index
value remains the same that is 0, but upper limit becomes
middle value - 1.Then step 2 will be repeated.
If the n is bigger than m, then you would find n in the second
half ,if the array is arranged in ascending order.Then you
dont want the first part anymore.discard it. So the length
changes after you discard the second part.Upper index value
remains the same, but lower limit becomes middle value +
1.Then step 2 will be repeated.
Example :
The array is
14 , 23, 28, 36, 69, 88
The number to be searched is 23.
Step 1:
Middle value = (lower index +Upper index)/2
= (0+5)/2
= 2.5
Either round off or truncate it. Let us take 3.So the middle value is 36.
Step 2 :
Compare 23 and 3 rd element 36.Both are not same.
Step 3 :
23 is smaller than 36, that means 23 will not be there after 36.
so discard second half, that is elements after 36.Lower index is still 0, and upper index becomes the value before the middle value , i.e 28.
Checking will go on till it finds the value.
If the value to be searched is 88 then,
88 is bigger than 36, therefore 88 will not be in the list
before 36.
so discard first half, that is elements before 36.upper
index remains the same and lower index becomes the
value after the middle value.
Checking will go on till it finds the value. Otherwise it will print the nmber not in the list.
class binary
{
public static void main(int a[],int n)---------------------------1
{
int l,i,mid,place,flag=0;
l=a.length;--------------------------------------------------------2
int low=0,up= l -1;----------------------------------------------3
while(low <= upp)----------------------------------------------4
{
mid = (low+up)/2;------------------------------------------5
if(a[mid]==n)
{
flag=1;------------------------------------------------6
place=mid;--------------------------------------------7
break;-------------------------------------------------8
}
else if(a[mid] < n)-----------------------------------------9
low=mid+1;
else
upp=mid - 1;
}
if(flag==1)
System.out.print(n+"is found at+(place+1));-----------------10
else
System.out.print(n+"is not found ");
}
}
step 1: input the array, number to be searched
step 2: find the length of the array
step 3:initialize the values for lower and upper index. l-1 coz, index starts from 0.
step 4:the loop should continue as long as lower limit is less than upper limit.
step 5:find the middle value.common mistake here is
(a[low]+a[upp])/2
step 6:there is some variable with some intial value so that when we find the number, change that value.If the value is not changed then it means the number is not found,coz you would not have gone to step 6.
step 7: if you want to find exactly where the number occurs in the array,then assign the mid index, not mid value.It is not a[mid].
step 8:Once we found the number,come out. no more searching.
step 9:check the number with middle value , change the low or upper index accordingly.
Isn't that easy.
Monday 28 July 2014
Thursday 24 July 2014
Class 7 revision
Write the full form for the following :
UTP
Wi – Fi
SMTP
CAN
HTTP
Answer in one word :
This is an empty tag.
Largest WAN in existence.
The largest heading tag.
New technology in wired transmission channels.
Choose the correct answer:
Which sequence of HTML tags are correct?
<html><head><title></title></head><body></body></html>
<html><head></head><body><title></<title></body></html>
<html><head><title></<title><body></body></head></html>
<html><title></<title><head></head><body"
Which of the following is the correct code to change the text to Calibri
WWW is based on which model?
3 D model
Client server model
Client model
None of the above
To display the text, which of the given tags is used
<centre>
<middle>
<center>
<length>
Use the words given below just once in order to fill in the gaps:
_____1____ is a computer that manages storage and retrieval of files. A network allows computers to ___2___, users to __3____ each other, a whole room of computers to ___4_____, etc. To set up a network you have to select an appropriate ___5___ to arrange the hardware devices using the media. Network connections between computers are typically created using __6_____. However, connections can be created using ____7___, telephone lines (and modems) or even, for very long distances, via ___8__links. Bluetooth is a __9____ technology designed for very ___10___ connections.
Differentiate between the following terms: [2x5=10]
Star Topology and Bus Topology
LAN and MAN
WYSIWYG editor and text editor
Client server architecture and Peer-to-Peer architecture
Answer the following questions:
What is networking ? Give two advantages.
What are the different components of network? Explain any one.
Sunday 20 July 2014
Photoshop Revision
Brush Palette
The user can select any type of brush from thousands of different types of brushes.
1. Foreground color
2. Background color
Layer Palette
The user can control the layers in the image by using the various buttons in the palette.
A. Layer visibility
B. Painting on the layer
C: Layer linked to active layer
D: New Layer
The user can select any type of brush from thousands of different types of brushes.
Channels
It is used to store information about the colour elements in an image.
Color Palette
It displays the foreground and background colors.
2. Background color
Layer Palette
The user can control the layers in the image by using the various buttons in the palette.
B. Painting on the layer
C: Layer linked to active layer
D: New Layer
Subscribe to:
Posts (Atom)