Monday, 22 September 2014
Sunday, 21 September 2014
Wednesday, 17 September 2014
Class 8 : Program
Visual Basic Programs
This is the same video I posted few days back, it was not very clear, I hope this is OK.
Tuesday, 16 September 2014
Saturday, 13 September 2014
Class 9 -Scanner
Input Method:
We are going to use Scanner for accepting values from the user . Its a simple one.
First include the package util.
import java.util.*;
Only small letters and * indicates all the files from the package and there is a semi colon in the end.
Then comes the main function
public static void main( )
{
Scanner S=new Scanner(System.in);
Remember S in red colour are capital letters. Here S is a variable can be in small or capital letter or any other variable name.
There are different statements for accepting different data type values.
int a= S.nextInt( );
float f= S.nextFloat( );
double x=S.nextDouble( );
But accepting character and string are different.
char ch=(char)System.in.read( );
String st=S.next( );
String st1=S.nextLine( );
next( ) accepts a word till a space and keeps the cursor in the same line.
nextLine( ) accepts words and moves the cursor to the next line after accepting the value.
Let us accept two numbers.
import java.util.*; ------------------------------------ 1
class ex1
{
public static void main( )
{
Scanner s=new Scanner(System.in); --------------- 2
int a, b,sum; --------------------------------------------- 3
System.out.println("Enter the first number"); ------ 4
a=s.nextInt( ); ------------------------------------------- 5
System.out.println("Enter the second number");----- 4
b=s.nextInt( );-------------------------------------------- 5
sum=a+b; -------------------------------------------------6
System.out.print("Total = " +sum);------------------- 7
}
}
1 is the first line in the program.
2 Write any variable instead of s , if you want.
3 is variable declaration.
4 is just a prompt message. A remainder to the user to enter a number.
5 Since I am accepting integer values, it should be nextInt. Remember no space after next and I in Int is capital.
6 is the calculation
7 is the output.
For other programs step 3 and step 5 will be different according to the data type you use.
We are going to use Scanner for accepting values from the user . Its a simple one.
First include the package util.
import java.util.*;
Only small letters and * indicates all the files from the package and there is a semi colon in the end.
Then comes the main function
public static void main( )
{
Scanner S=new Scanner(System.in);
Remember S in red colour are capital letters. Here S is a variable can be in small or capital letter or any other variable name.
There are different statements for accepting different data type values.
int a= S.nextInt( );
float f= S.nextFloat( );
double x=S.nextDouble( );
But accepting character and string are different.
char ch=(char)System.in.read( );
String st=S.next( );
String st1=S.nextLine( );
next( ) accepts a word till a space and keeps the cursor in the same line.
nextLine( ) accepts words and moves the cursor to the next line after accepting the value.
Let us accept two numbers.
import java.util.*; ------------------------------------ 1
class ex1
{
public static void main( )
{
Scanner s=new Scanner(System.in); --------------- 2
int a, b,sum; --------------------------------------------- 3
System.out.println("Enter the first number"); ------ 4
a=s.nextInt( ); ------------------------------------------- 5
System.out.println("Enter the second number");----- 4
b=s.nextInt( );-------------------------------------------- 5
sum=a+b; -------------------------------------------------6
System.out.print("Total = " +sum);------------------- 7
}
}
1 is the first line in the program.
2 Write any variable instead of s , if you want.
3 is variable declaration.
4 is just a prompt message. A remainder to the user to enter a number.
5 Since I am accepting integer values, it should be nextInt. Remember no space after next and I in Int is capital.
6 is the calculation
7 is the output.
For other programs step 3 and step 5 will be different according to the data type you use.
Tuesday, 9 September 2014
Class 8
Declaring a variable
The first line in the program would be variable declaration. It includes the Dim statement , name of the variable and the data type.
Dim age as integer
Dim name as String
If you have more than one variable,
Dim a,b,c as Integer (same data type)
Dim x,y as Integer, i,j as Double (different data types)
Now the second step.
We need to give values for those variables.
Dim a as Integer = 100
or
Dim A as Integer
A=10
Activity
1. Declare the integer variables x,y and z
Dim x,y,z as Integer
2. Assign values 5 to x, 10 to y and 15 to z
x=5
y=10
z=15
3. What are the values of C, N1, N2, N3 :
A=5
B=10
C=A+B
N1=A+10
N2=B
N3=5+N2
C=15( since A is 5 and B is 10)
N1=15 ( A is 5 and add 10 to it)
N2=10(B is 10)
N3=15 (N2 is 10 and add 5 to it)
Sring Functions
1. Print Len("Cotton Boys)
11(including space)
2. Print Left("BCBS", 2)
BC
3. Print Right("Bangalore",4)
lore
4. Print Mid("Sesquicentennial",7,4)
cent
5. Print Ucase("Sony")
SONY
6. Print Lcase("Computer SCIENCE")
computer science
7. Print Instr("Happiness","in")
5 (5 is the place value of i)
Print Instr("Happiness","at")
0 (since there is no at in the string Happiness)
8. Print val("40") + val("20")
60
Print ("40") + ("60")
4060( since both are NOT numbers but strings, so they are
joined together not added)
9. Print Replace("World/Wide","/","_")
World_Wide(/ is replaced with _)
The first line in the program would be variable declaration. It includes the Dim statement , name of the variable and the data type.
Dim age as integer
Dim name as String
If you have more than one variable,
Dim a,b,c as Integer (same data type)
Dim x,y as Integer, i,j as Double (different data types)
Now the second step.
We need to give values for those variables.
Dim a as Integer = 100
or
Dim A as Integer
A=10
Activity
1. Declare the integer variables x,y and z
Dim x,y,z as Integer
2. Assign values 5 to x, 10 to y and 15 to z
x=5
y=10
z=15
3. What are the values of C, N1, N2, N3 :
A=5
B=10
C=A+B
N1=A+10
N2=B
N3=5+N2
C=15( since A is 5 and B is 10)
N1=15 ( A is 5 and add 10 to it)
N2=10(B is 10)
N3=15 (N2 is 10 and add 5 to it)
Sring Functions
1. Print Len("Cotton Boys)
11(including space)
2. Print Left("BCBS", 2)
BC
3. Print Right("Bangalore",4)
lore
4. Print Mid("Sesquicentennial",7,4)
cent
5. Print Ucase("Sony")
SONY
6. Print Lcase("Computer SCIENCE")
computer science
7. Print Instr("Happiness","in")
5 (5 is the place value of i)
Print Instr("Happiness","at")
0 (since there is no at in the string Happiness)
8. Print val("40") + val("20")
60
Print ("40") + ("60")
4060( since both are NOT numbers but strings, so they are
joined together not added)
9. Print Replace("World/Wide","/","_")
World_Wide(/ is replaced with _)
Monday, 8 September 2014
Class 7 - Hyperlink
Write a complete HTML program to create a webpage as given below.
The title of the page is an image file (title.png) , and the
other image file is gladiator.jpg.
other image file is gladiator.jpg.
The file names of different notepad files are histry.html, image1.html, image2.html, image3.html, image4.html. Link all the pages.Create only the main file.
Now you have to create only the main file.
<html>
<head>
<title> Cottonian Shield</title>
</head>
<body>
<center><img src=title.png></center> <br>
<center><img src=gladiator.jpg></center><br>
<a href=histry.html>History</a><br>
<a href=image1.html>Image 1</a><br>
<a href=image2.html>Image 2</a><br>
<a href=image3.html>Image 3</a><br>
<a href=image4.html>Image 4</a><br>
</body>
</html>
Remember after <a href write the file name if it is given in the question or else assume some name. Do not write the picture name there, write only the notepad file name, close the bracket. Then write the hypertext that is the text which on clicking will take you to the given page . In the example given below the hypertexts are History,Image 1,Image2,Image3,Image 4.
By the way those are my boys Jeswanth, Vinay and Sapthagiri (8 B last year). Now they are in senior team.
Now you have to create only the main file.
<html>
<head>
<title> Cottonian Shield</title>
</head>
<body>
<center><img src=title.png></center> <br>
<center><img src=gladiator.jpg></center><br>
<a href=histry.html>History</a><br>
<a href=image1.html>Image 1</a><br>
<a href=image2.html>Image 2</a><br>
<a href=image3.html>Image 3</a><br>
<a href=image4.html>Image 4</a><br>
</body>
</html>
Remember after <a href write the file name if it is given in the question or else assume some name. Do not write the picture name there, write only the notepad file name, close the bracket. Then write the hypertext that is the text which on clicking will take you to the given page . In the example given below the hypertexts are History,Image 1,Image2,Image3,Image 4.
By the way those are my boys Jeswanth, Vinay and Sapthagiri (8 B last year). Now they are in senior team.
Class 7 - Revision(HTML)
Select the correct answer:
2. Container element has both
14. The text between <title> and </title> will be displayed
1. Write a program in HTML to display the following :
My first program ---------------------------- 1
I am in class 7 -----------------------------2
Bishop Cotton Boys' School -----------------------------3
<html>
<head>
<title> Example 1</title>
</head>
<body >
<b> My first program</b> <br>
I am in <I> class 7 </I> <p>
<u> Bishop Cotton Boys' School</p>
</body>
</html>
The line1 is darker than the rest of the program. This is because of the bold tag. to go to the next line use the <br> tag.
In line 2 , only class 7 is slanting , so use italics tag for that.
There is a blank line between line 2 and line 3, so paragraph tag should be used. Even if you close <p> before <u> tag also will give same effect.
In line 3,a line is drawn below the whole sentence using <u> tag.
2. What would be the output for the following program:
<html>
<head>
<title> Example 2</title>
</head>
<body bgcolor=olive > ------------------1
<font color=white size=6 face= Mistral> ------------------2
Computers<br>Class 7
<p>
Cottonnian shield...................
</p>
<!- I am going to draw a line - > -----------------3
<hr size=5 > -----------------4
</font>
</body>
</html>
Line 1 : the background colour. The spelling is bgcolor.
Line 2 : font color makes the text and numbers to turn to that particular colour. face changes the default font name to the name given.
Line 3 : This is the comment line , which will not be displayed in the web browser.
Line 4: The horizontal line with size 5
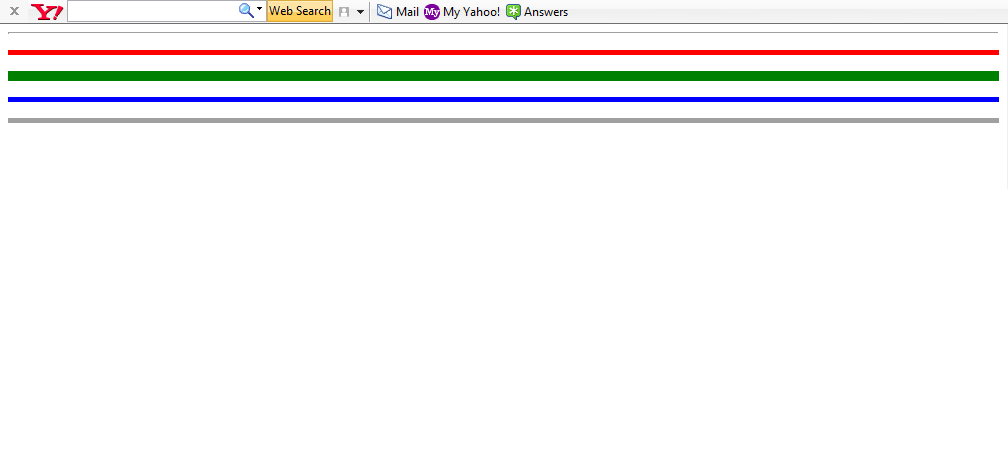
The above output has 5 lines. The first one is a thin line, because there is no size tag.
<html>
<head>
<title> Example 3</title>
</head>
<body>
<hr > <p>
<hr size=3 color=red> <p>
<hr size=8 color=green></p>
<hr size=3 align=right color=blue><p>
<hr size=3 noshade ></p>
</body>
</html>
Image
<img src=filename>
<img src= bird.jpg>
Attributes of image tag :
1. width - changes the width of the image
2. height - changes the height of the image
3. border - border around the image, default value is 0.
4. alt - if the image is not available, the alternate text will be displayed.
5. align - position of the image in the web page.
align =right/left/middle/top/bottom
<html>
<body>
<img src=moon.jpg height=400 width=400>
</body>
</html>
- HTML stands for
- Hyper Text Markup Language
- Hyper Text Makeup Language
2. Container element has both
- opening and middle tag
- opening and closing tag
- a line break tag
- an empty tag
- line break and an empty tag
- an editor
- a web browser
- search engine
- <body backcolor=red>
- <body bgcolour=red>
- <body bgcolor=red>
- <body backcolour=red>
- <body bg color=red>
- <text=green>
- <body textcolour=green>
- <body text=green>
- <textcolour=green>
- moves the cursor to the next line
- leaves a blank line
- leaves a paragraph
- comments
- title
- body
- <align=center>
- <align=centre>
- <p align=centre>
- <center>
- 1 to 7
- 1 to 6
- 1 to 3
- <dark>
- <b>
- <bold>
- <u>
- <line>
- <strike>
- <strikethrough>
- <head>
- <h1>
- <h6>
14. The text between <title> and </title> will be displayed
- in the title bar
- in the heading bar
- in the web page
- none of the above
- an additional information about the tag
- main tag
- an example
- MS Word
- Frontpage
- HTML
1. Write a program in HTML to display the following :
My first program ---------------------------- 1
I am in class 7 -----------------------------2
Bishop Cotton Boys' School -----------------------------3
<html>
<head>
<title> Example 1</title>
</head>
<body >
<b> My first program</b> <br>
I am in <I> class 7 </I> <p>
<u> Bishop Cotton Boys' School</p>
</body>
</html>
The line1 is darker than the rest of the program. This is because of the bold tag. to go to the next line use the <br> tag.
In line 2 , only class 7 is slanting , so use italics tag for that.
There is a blank line between line 2 and line 3, so paragraph tag should be used. Even if you close <p> before <u> tag also will give same effect.
In line 3,a line is drawn below the whole sentence using <u> tag.
2. What would be the output for the following program:
<html>
<head>
<title> Example 2</title>
</head>
<body bgcolor=olive > ------------------1
<font color=white size=6 face= Mistral> ------------------2
Computers<br>Class 7
<p>
Cottonnian shield...................
</p>
<!- I am going to draw a line - > -----------------3
<hr size=5 > -----------------4
</font>
</body>
</html>
Line 1 : the background colour. The spelling is bgcolor.
Line 2 : font color makes the text and numbers to turn to that particular colour. face changes the default font name to the name given.
Line 3 : This is the comment line , which will not be displayed in the web browser.
Line 4: The horizontal line with size 5
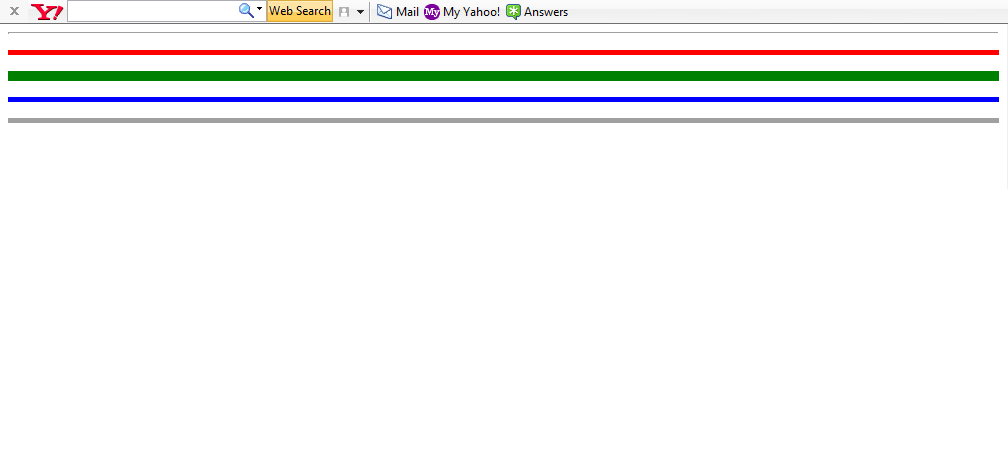
The above output has 5 lines. The first one is a thin line, because there is no size tag.
<html>
<head>
<title> Example 3</title>
</head>
<body>
<hr > <p>
<hr size=3 color=red> <p>
<hr size=8 color=green></p>
<hr size=3 align=right color=blue><p>
<hr size=3 noshade ></p>
</body>
</html>
Image
<img src=filename>
<img src= bird.jpg>
Attributes of image tag :
1. width - changes the width of the image
2. height - changes the height of the image
3. border - border around the image, default value is 0.
4. alt - if the image is not available, the alternate text will be displayed.
5. align - position of the image in the web page.
align =right/left/middle/top/bottom
<html>
<body>
<img src=moon.jpg height=400 width=400>
</body>
</html>
Subscribe to:
Posts (Atom)